- Inner Join In R Dplyr
- How To Merge Rows In R
- Inner Join In R On Two Columns
- Inner Join In R On Two Columns
R/join.r
These are generic functions that dispatch to individual tbl methods - see themethod documentation for details of individual data sources. x
andy
should usually be from the same data source, but if copy
isTRUE
, y
will automatically be copied to the same source as x
.
Arguments
x, y | tbls to join |
---|---|
by | a character vector of variables to join by. If To join by different variables on x and y use a named vector.For example, |
copy | If |
suffix | If there are non-joined duplicate variables in |
... | other parameters passed onto methods, for instance, |
keep | If |
name | the name of the list column nesting joins create. If |
In this video I'm showing you how to merge data frames with the dplyr package in R. The video includes six different join functions, i.e. Innerjoin, leftjo. In a World Without anti-joins. Let's first try to figure this out without the use of anti-joins. Based on what we know now around left joins we could do the following: accounts%% leftjoin(activity, by = 'accountid')%% filter(is.na(activitytype)) As you can see, we can left join the activity dataset to our accounts dataset. Note: The INNER JOIN keyword selects all rows from both tables as long as there is a match between the columns. If there are records in the 'Orders' table that do not have matches in 'Customers', these orders will not be shown! An inner join keeps observations that appear in both tables. An outer join keeps observations that appear in at least one of the tables. There are three types of outer joins: A left join keeps all observations in x. A right join keeps all observations in y. A full join keeps all observations in x and y.
Join types
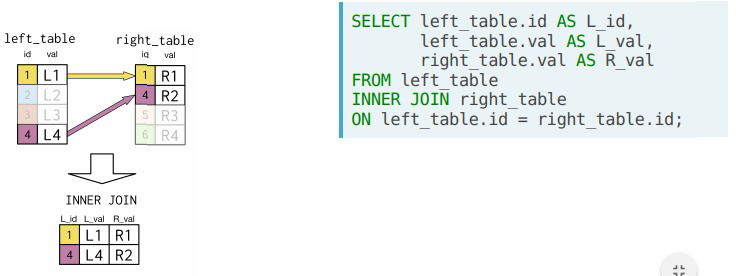
Currently dplyr supports four types of mutating joins, two types of filtering joins, anda nesting join.
Mutating joins combine variables from the two data.frames:
inner_join()
return all rows from x
where there are matchingvalues in y
, and all columns from x
and y
. If there are multiple matchesbetween x
and y
, all combination of the matches are returned.
left_join()
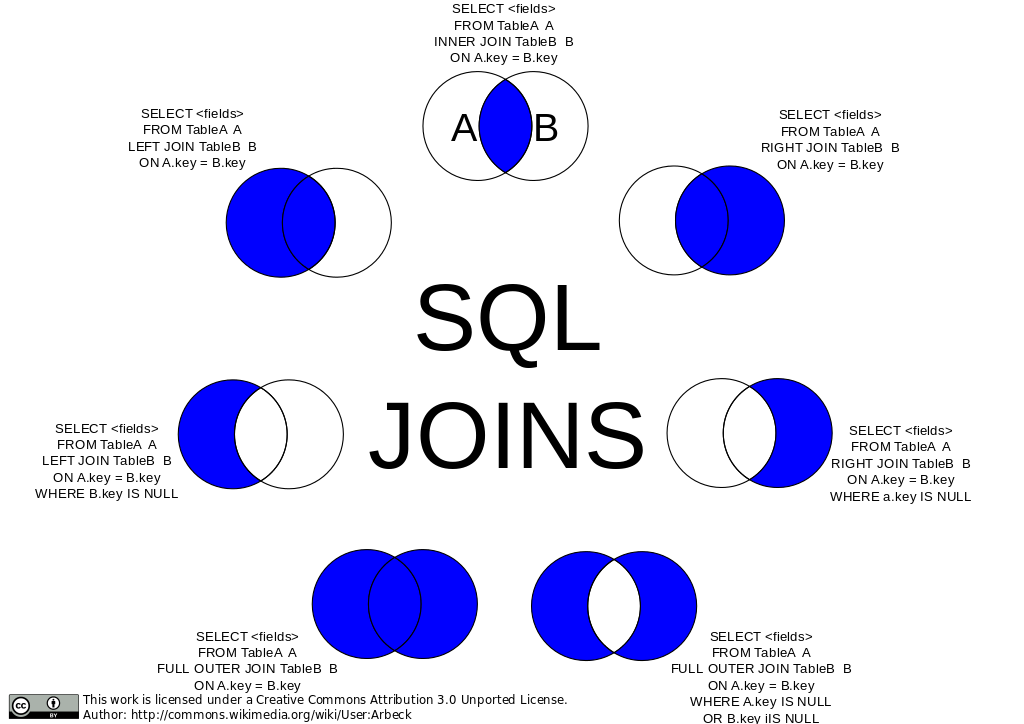
Inner Join In R Dplyr
return all rows from x
, and all columns from x
and y
. Rows in x
with no match in y
will have NA
values in the newcolumns. If there are multiple matches between x
and y
, all combinationsof the matches are returned.
right_join()
return all rows from y
, and all columns from x
and y. Rows in y
with no match in x
will have NA
values in the newcolumns. If there are multiple matches between x
and y
, all combinationsof the matches are returned.
full_join()
return all rows and all columns from both x
and y
.Where there are not matching values, returns NA
for the one missing.
Filtering joins keep cases from the left-hand data.frame:
semi_join()
return all rows from x
where there are matchingvalues in y
, keeping just columns from x
. A semi join differs from an inner join because an inner join will returnone row of x
for each matching row of y
, where a semijoin will never duplicate rows of x
.
anti_join()
return all rows from x
where there are notmatching values in y
, keeping just columns from x
.
Nesting joins create a list column of data.frames:
nest_join()
return all rows and all columns from x
. Adds alist column of tibbles. Each tibble contains all the rows from y
that match that row of x
. When there is no match, the list column isa 0-row tibble with the same column names and types as y
. nest_join()
is the most fundamental join since you can recreate the other joins from it.An inner_join()
is a nest_join()
plus an tidyr::unnest()
, and left_join()
is anest_join()
plus an unnest(.drop = FALSE)
.A semi_join()
is a nest_join()
plus a filter()
where you check that every element of data hasat least one row, and an anti_join()
is a nest_join()
plus a filter()
where you check every element has zero rows.
How To Merge Rows In R
Grouping
Groups are ignored for the purpose of joining, but the result preservesthe grouping of x
.
Examples
If you browse through our technical blog posts you'll see quite a few devoted to the data analysis functionality in the R packge dplyr
. This is due to the fact that we are constantly finding fun new functions to play with. We wanted to devote this small post to an unexpectedly useful function called anti_join
.
Using anti_join()
from the dplyr
package
For most data analysis tasks you may have two tables you want to join based on a common ID. This is straightforward in any data analysis package. But occasionally, especially in quality assurance types of settings, we find ourselves wanting to identify the records from one table that did NOT match the other table. For example, anti_join
came in handy for us in a setting where we were trying to re-create an old table from the source data. We then wanted to be able to identify the records from the original table that did not exist in our updated table. This is where anti_join
comes in, especially when you're dealing with a multi-column ID.
Inner Join In R On Two Columns
We'll start with a relatively simple example.
Inner Join In R On Two Columns
To identify the rows that exist in table1 but not in table2 you could use any number of strategies:
You might ask why anti_join
is an advance given the other easy solutions we're showing above. We find it most useful when our common ID is a combination of multiple columns. So let's use another example where we have a multi-column common ID:
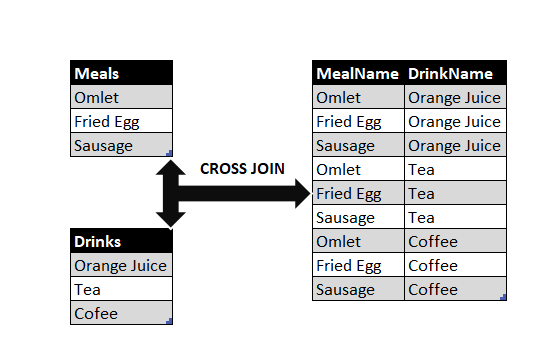
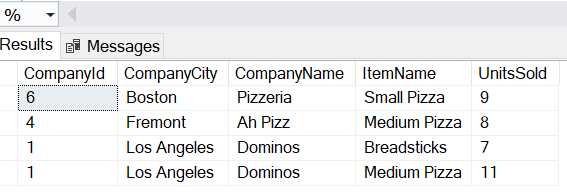
Currently dplyr supports four types of mutating joins, two types of filtering joins, anda nesting join.
Mutating joins combine variables from the two data.frames:
inner_join()
return all rows from x
where there are matchingvalues in y
, and all columns from x
and y
. If there are multiple matchesbetween x
and y
, all combination of the matches are returned.
left_join()
Inner Join In R Dplyr
return all rows from x
, and all columns from x
and y
. Rows in x
with no match in y
will have NA
values in the newcolumns. If there are multiple matches between x
and y
, all combinationsof the matches are returned.
right_join()
return all rows from y
, and all columns from x
and y. Rows in y
with no match in x
will have NA
values in the newcolumns. If there are multiple matches between x
and y
, all combinationsof the matches are returned.
full_join()
return all rows and all columns from both x
and y
.Where there are not matching values, returns NA
for the one missing.
Filtering joins keep cases from the left-hand data.frame:
semi_join()
return all rows from x
where there are matchingvalues in y
, keeping just columns from x
. A semi join differs from an inner join because an inner join will returnone row of x
for each matching row of y
, where a semijoin will never duplicate rows of x
.
anti_join()
return all rows from x
where there are notmatching values in y
, keeping just columns from x
.
Nesting joins create a list column of data.frames:
nest_join()
return all rows and all columns from x
. Adds alist column of tibbles. Each tibble contains all the rows from y
that match that row of x
. When there is no match, the list column isa 0-row tibble with the same column names and types as y
. nest_join()
is the most fundamental join since you can recreate the other joins from it.An inner_join()
is a nest_join()
plus an tidyr::unnest()
, and left_join()
is anest_join()
plus an unnest(.drop = FALSE)
.A semi_join()
is a nest_join()
plus a filter()
where you check that every element of data hasat least one row, and an anti_join()
is a nest_join()
plus a filter()
where you check every element has zero rows.
How To Merge Rows In R
Grouping
Groups are ignored for the purpose of joining, but the result preservesthe grouping of x
.
Examples
If you browse through our technical blog posts you'll see quite a few devoted to the data analysis functionality in the R packge dplyr
. This is due to the fact that we are constantly finding fun new functions to play with. We wanted to devote this small post to an unexpectedly useful function called anti_join
.
Using anti_join()
from the dplyr
package
For most data analysis tasks you may have two tables you want to join based on a common ID. This is straightforward in any data analysis package. But occasionally, especially in quality assurance types of settings, we find ourselves wanting to identify the records from one table that did NOT match the other table. For example, anti_join
came in handy for us in a setting where we were trying to re-create an old table from the source data. We then wanted to be able to identify the records from the original table that did not exist in our updated table. This is where anti_join
comes in, especially when you're dealing with a multi-column ID.
Inner Join In R On Two Columns
We'll start with a relatively simple example.
Inner Join In R On Two Columns
To identify the rows that exist in table1 but not in table2 you could use any number of strategies:
You might ask why anti_join
is an advance given the other easy solutions we're showing above. We find it most useful when our common ID is a combination of multiple columns. So let's use another example where we have a multi-column common ID:
With a two-column unique ID using %in%
or match()
is more challenging. You could create a single ID by concatenating the state/county fields but this adds a messy extra step. Instead anti_join() is your savior: